Vue Dropdown with Image: A Vue dropdown with image is a UI component that allows users to select from a list of options presented in a dropdown menu. This type of dropdown includes an image or icon next to each option to provide visual cues and enhance user experience. The Vue framework offers several libraries and plugins for building custom dropdowns, including Vuetify and Bootstrap Vue. When designing a Vue dropdown with image, developers should consider accessibility, responsiveness, and user feedback to ensure the component is intuitive and easy to use. Overall, a Vue dropdown with image can be a useful tool for improving the usability and aesthetics of web applications.
How can I create a dropdown menu in Vue that includes images for each option?
This code creates a Vue app with a dropdown menu that includes images for each option. It uses a data object to store the dropdown state and options, including their labels and images. The created
method sets the first option as the initially selected option.
The template includes a div with a class of dropdown
that contains a dropdown toggle and a dropdown menu. The toggle displays the selected option’s label and image, and the menu shows all available options with their labels and images.
The toggle and menu are bound to isDropdownOpen
, which toggles the dropdown’s visibility when clicked. The selectOption
method updates the selected option and closes the dropdown menu when an option is clicked.
The code uses Vue directives and binding to render the options dynamically and bind event listeners. Overall, this code provides a simple and functional implementation of a Vue dropdown with images
Vue Dropdown with Image Example
<div id="app">
<div class="dropdown">
<div class="dropdown-toggle" v-on:click="toggleDropdown">
<img :src="selectedOption.image" class="dropdown-option-image">
<span class="dropdown-option-label">{{ selectedOption.label }}</span>
<span class="dropdown-caret"></span>
</div>
<ul class="dropdown-menu" v-show="isDropdownOpen">
<li v-for="option in options" v-on:click="selectOption(option)">
<img :src="option.image" class="dropdown-option-image">
<span class="dropdown-option-label">{{ option.label }}</span>
</li>
</ul>
</div>
</div>
<script type="module">
const app = Vue.createApp({
data() {
return {
isDropdownOpen: false,
selectedOption: null,
options: [
{ label: 'John Andrew ', image: 'https://pixabay.com/get/gc038694352a3c2d81de005870a75bb5ff6f54063b4e978eae234119fbf99725c4e1b34860a1d1f5733cf9d319417f157_640.jpg' },
{ label: 'Mr. Bob', image: 'https://pixabay.com/get/g5fb5c90665d60f5a94f29e8590ac965c13f4eee6cd191e3845ccdf0ac0e2c842ca7ff998e6bc9beb03e0e63a1408080215eed58421213a40f810c07d6f228c0b_640.jpg' },
{ label: 'Jimmy Charlie', image: 'https://pixabay.com/get/g9bd25111789b38bb87c9c6d43f72f500138e6907d85d9163aea2800590c0a464a8657635d97cb7da9040b4c70fa43d15_640.jpg' },
{ label: 'David', image: 'https://pixabay.com/get/g5515a925fc14b6cf145264d7556e6fd066ba96dae89c1c8b919ae237d614ded78b10794e80ad195ff4628875cae4d5a9_640.jpg' },
],
};
},
created() {
this.selectedOption = this.options[0];
},
methods: {
toggleDropdown() {
this.isDropdownOpen = !this.isDropdownOpen;
},
selectOption(option) {
this.selectedOption = option;
this.isDropdownOpen = false;
},
},
});app.mount('#app');
</script>
Output of Vue Dropdown with Image
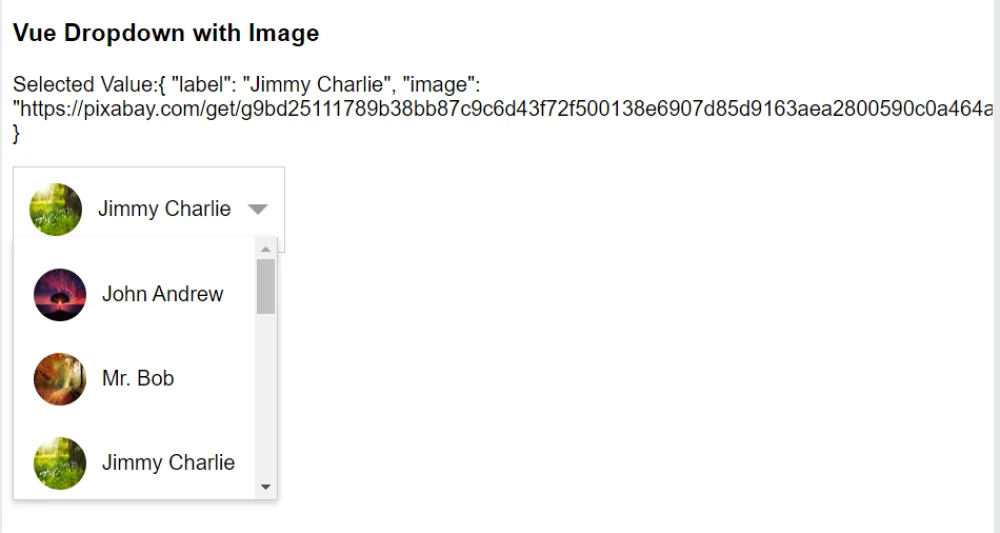